FM Radio
About 1 min
FM Reception Decoding
For the FM reception decoding part, the following GRC design is used:
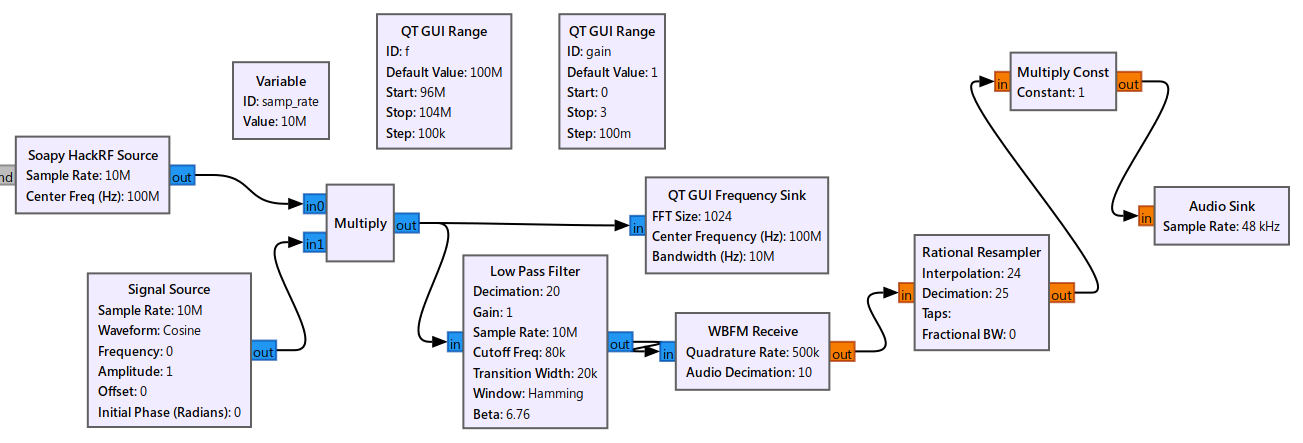
Module parameters:
- Soapy HackRF: Center Freq=100e6
- Range f=96e6~104e6
- Signal Source: Freq=100e6-f
- Low Pass Filter: Decimation=20, Cutoff Freq=80e3, Transition_width=20e3
- WBFM Receive: Quadrature Rate=500e3, Audio Decimation=10
- Rational Resampler: Decimation=25, Interpolation=24
- Range gain=0~3
- Audio Sink: 48e3
Set the ID as fm_recv, and set the saved file as fm_recv.py.
FM Control Panel
We slightly modified the Panel panel from the previous page as follows:
import sys
from PyQt5 import Qt
class Station(Qt.QWidget):
stations = {
"ICRT(100.7)": 100.7,
"Wind City Radio(98.7)": 98.7,
"International Broadcasting Station(101.1)": 101.1,
}
def __init__(self, grc):
super().__init__() # parent initialization
self.setWindowTitle("Radio Station") # set title
self.grc = grc # save the grc instance
vlayout = Qt.QVBoxLayout(self) # create a vertical layout
self.setLayout(vlayout) # set the layout
for name, freq in self.stations.items(): # loop items
btn = Qt.QRadioButton(name) # add a button
btn.toggled.connect(self.changeFreq) # connect click event
vlayout.addWidget(btn) # put into the vlayout
def changeFreq(self): # event handler
btn = self.sender() # get sender button
freq = float(self.stations[btn.text()])
self.grc.set_f(freq*1e6) # set frequency
The modifications made here include:
- Changing the radio channel list, which is reset based on the current situation.
- In the
__init__
function, we pass the object generated by GNU Radio and set it as the self.grc attribute. - In the
changeFreq
function, we pass the obtained frequency back to the setting function of self.grc.
Main Program
Write a main program main.py
to combine the above two program modules together:
import sys
from PyQt5 import Qt
from fm_recv import fm_recv
from station import Station
qapp = Qt.QApplication(sys.argv)
grc = fm_recv()
grc.start()
grc.show()
fmStation = Station(grc)
fmStation.show()
qapp.exec_()
Execution result:
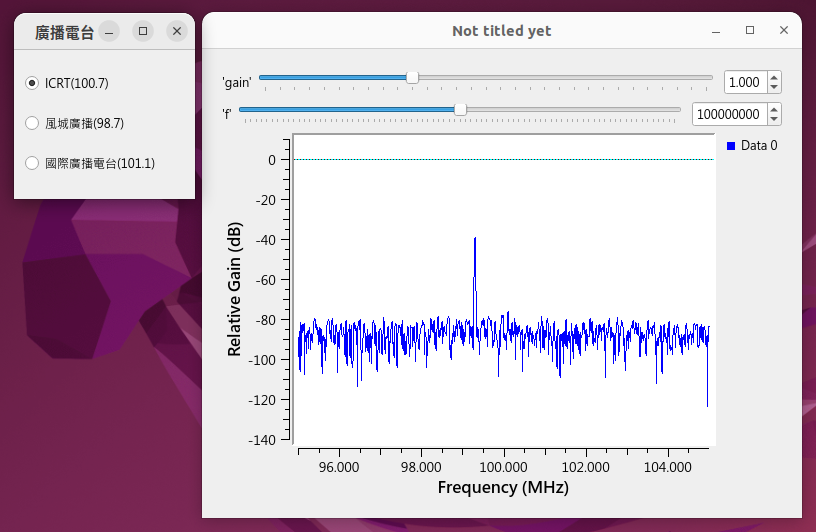
Exercise 4
- Execute the above code to ensure it works correctly.
- Add more radio stations or change the layout.