Drawing Fourier Series
Drawing Fourier Series
Assuming the function is defined on the interval , it was mentioned earlier that can be represented as the sum of its Fourier series:
where
and are the harmonic coefficients.
Next, we will use Octave to observe a sawtooth wave, calculate the coefficients of its Fourier series, then sum up the components of harmonics and compare it with the original waveform. The wave function used here is as follows:
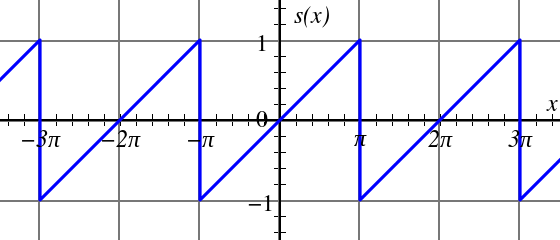
Its Fourier series can be calculated as:
The steps of the program are as follows:
MATLAB has a
sawtooth
function that can directly draw a sawtooth waveform. Thesawtooth
function is defined in the Signal Processing Toolbox. If using MATLAB, ensure this toolbox is correctly installed; if using Octave, then thesignal
package can be used. For installing Octave packages in Windows, refer to the official website. For Ubuntu, use the following command to install:sudo apt-get install octave-signal
In Octave, first use the
pkg load signal
command to load the signal package. Then, you can use thesawtooth
function (usehelp sawtooth
to view the usage of this function). Try using the following command to draw a sawtooth function graph:t = -8:0.1:8; s = sawtooth(t-pi); % sawtooth's default period is 2*pi, with a turn at 0 plot(t,s); grid on; % draw grid lines
Observe if the waveform is correct.
Draw a partial sum of the series.
N = 5; % number of terms sgn = 1; % used for alternating signs, the first term is positive t = -8:0.1:8; fs = zeros(size(t)); % set fs to the same dimension as t, all zeros for n = 1:N % from 1 to N fs = fs + 2*sgn*sin(n*t)/(n*pi); % add one term sgn = sgn * -1; % change sign end plot(t,fs); % plot the graph
Observe the synthesized waveform.
Finally, plot both waveforms together, as shown in the following code:
N = 5; t = -8:0.1:8; sgn = 1; s = sawtooth(t-pi); % sawtooth's default period is 2 pi, with a turn at 0 fs = zeros(size(s)); for n = 1:N fs = fs + 2*sgn*sin(n*t)/(n*pi); sgn = sgn * -1; end plot(t,s,'b',t,fs,'r'); grid on; % draw grid lines
The result after execution is as follows:
In the above program, we already know the coefficients of and for the Fourier series of . For other functions, it might take a lot of time to calculate. In practice, Octave has a Symbolic Math Toolbox that can assist in symbolic operations. If using Octave, install the symbolic
package with the following command:
sudo apt-get install octave-symbolic
Then, use pkg load symbolic
to load the package for use. For example, for , assuming it's defined in the interval , you can calculate the coefficients as follows:
pkg load symbolic
syms x n; % x, n as symbols
f = x^2; % f(x) = x^2
an = int(f*cos(n*x), x, -pi, pi) / pi; % calculate the definite integral value
Exercise 3
Octave has a square
function that can be used to draw square wave functions (check with help square
). Please calculate the Fourier series of a square wave (you can also look it up online or use the symbolic package for calculation), then use a program to draw the square wave and its partial Fourier series sum. You can define the period and amplitude of the square wave yourself.