Python 取樣
大约 1 分鐘
Python 有很多套件可用,其中對於數據處理的部份,numpy 和 matplotlib 都是常被使用的套件,前者可用來處理各種矩陣運算,後者可以用來畫圖,這兩個合起來,可以執行很多 MATLAB/Octave 程式的功能,而且語法非常相似。
我們一樣假設信號頻率為9,然後分別用取樣頻率100和10來觀察信號取樣的結果。程式碼如下:
import numpy as np
import matplotlib.pyplot as plt
# Define the time vector for one second duration
t = np.arange(0, 1, 0.001) # Time from 0 to 1 second with 1ms interval
# Define the frequency of the cosine wave
f = 9 # Frequency of 9 Hz
# Generate the cosine wave
cosine_wave = np.cos(2 * np.pi * f * t)
# Plot the original cosine wave
plt.figure()
plt.subplot(3, 1, 1)
plt.plot(t, cosine_wave)
plt.title('Original Cosine Wave (9 Hz)')
# Sample the cosine wave at 100 Hz
fs1 = 100 # Sampling rate of 100 Hz
n1 = np.arange(0, 1, 1/fs1) # Sample times
samples1 = np.cos(2 * np.pi * f * n1)
# Plot the sampled signal at 100 Hz
plt.subplot(3, 1, 2)
plt.stem(n1, samples1, 'r', markerfmt='ro', basefmt=" ")
plt.title('Sampled at 100 Hz')
# Sample the cosine wave at 10 Hz
fs2 = 10 # Sampling rate of 10 Hz
n2 = np.arange(0, 1, 1/fs2) # Sample times
samples2 = np.cos(2 * np.pi * f * n2)
# Plot the sampled signal at 10 Hz
plt.subplot(3, 1, 3)
plt.stem(n2, samples2, 'g', markerfmt='go', basefmt=" ")
plt.title('Sampled at 10 Hz')
# Show the results
plt.xlabel('Time (seconds)')
plt.ylabel('Amplitude')
plt.tight_layout()
plt.show()
執行結果如下:
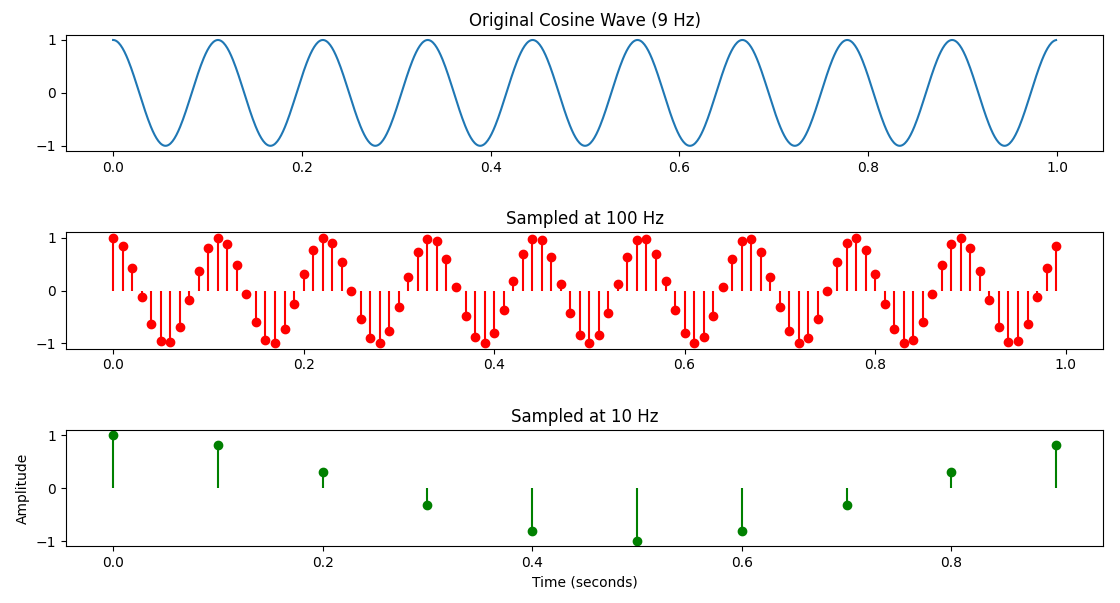
可以看到不管程式碼,或者執行的結果,都和 Octave 的部份非常相似。
練習 4
練習安裝上面所說的兩個套件,並實際執行上述的程式碼,觀察結果是否相同。(建議用 Anaconda 或 venv 建立一個 Python 的執行環境,並用 pip install numpy matplotlib
安裝套件)